All about Building rest apis with flask
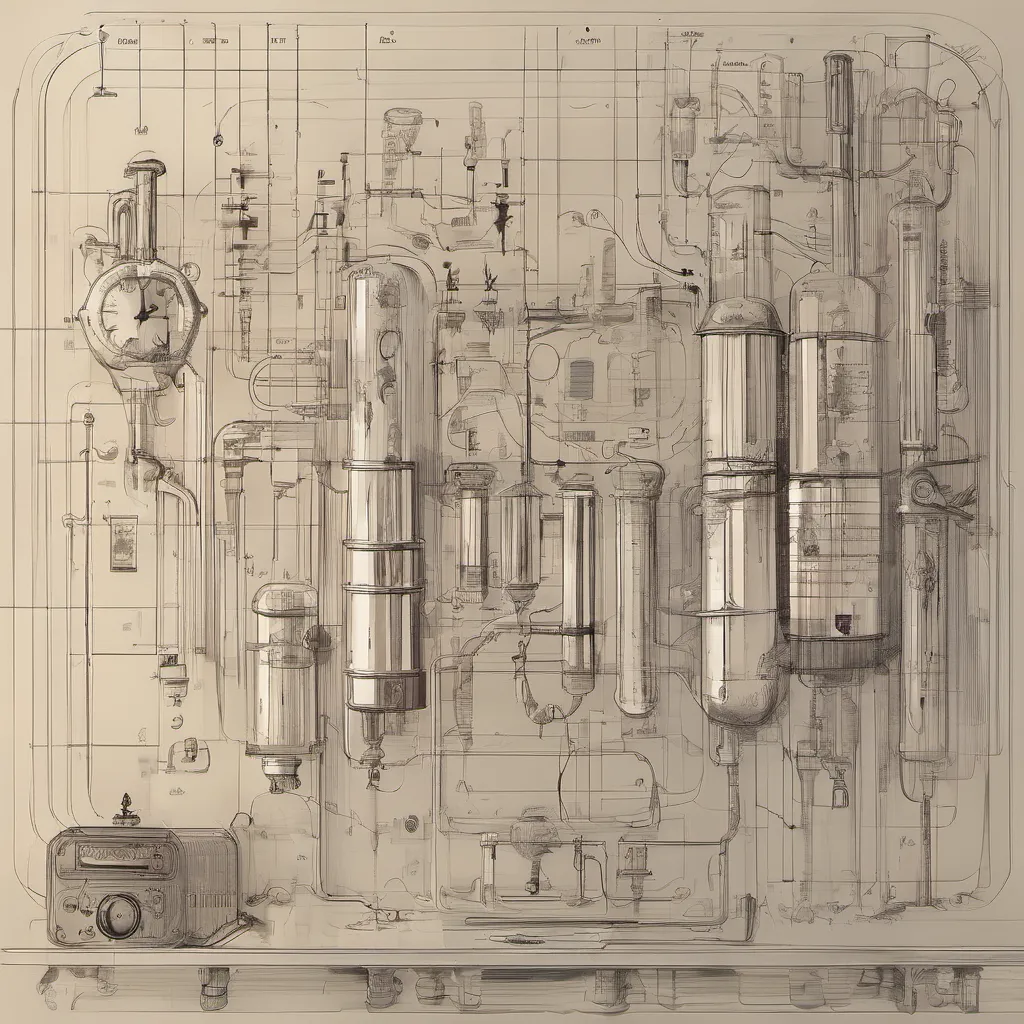
Building REST APIs with Flask: A Step-by-Step Guide
Flask is a popular Python web framework that makes it easy to build robust and efficient web applications. One of its key features is the ability to create RESTful APIs, which are used for data exchange between client-server systems. In this article, we'll explore how to build REST APIs with Flask.Why Use Flask?
Flask is a lightweight framework that allows you to focus on building your application without being bogged down by unnecessary features.
Setting Up Your Project
To get started, create a new project using pip:
1:
pip install flask
- Install Flask
Defining Routes
In Flask, you define routes to map HTTP requests to specific functions. Use the @app.route decorator to specify the URL and method for a route:
```python from flask import Flask app = Flask(__name__) @app.route('/hello', methods=['GET']) def hello_world(): return 'Hello World!' ```Handling Requests
To handle requests, use the request object provided by Flask. This object contains information about the current request:
```python from flask import request @app.route('/users', methods=['GET']) def get_users(): users = [{'id': 1}, {'id': 2}] return jsonify(users) ```Error Handling
When handling requests, you'll likely encounter errors. Use try-except blocks to catch and handle exceptions:
```python try: # Code that might raise an exception except Exception as e: return {'error': str(e)}, 500 ```Testing Your API
Use tools like Postman or curl to test your API. You can also write unit tests using a testing framework like pytest:
1:
pip install pytest
Conclusion
Building REST APIs with Flask is a straightforward process that requires attention to detail and proper error handling. With the steps outlined in this guide, you can create robust and efficient web applications using Flask.
We hope this article has been helpful! Let us know if you have any questions or need further assistance.
Files in This Knowledge Base
Experiential AI content created by David Beck.
Basics of python programming
Building rest apis with flask
Data structures in python
Machine learning basics in python
Python libraries for data analysis (pandas, numpy)
Real world python projects
Testing and debugging python applications
Version control using git
Web scraping with beautifulsoup and selenium
Working with databases (sqlalchemy, sqlite)
Writing clean, modular code
View Other Knowledge Bases
Contact Me
07748311327








#DavidWilliamBeck #DigitalMarketingExecutive #WebsiteDeveloper #Marketing #CommunityManager #Python #YouTuber #David #William #Beck #DevLife #SocialMedia #Wartorious